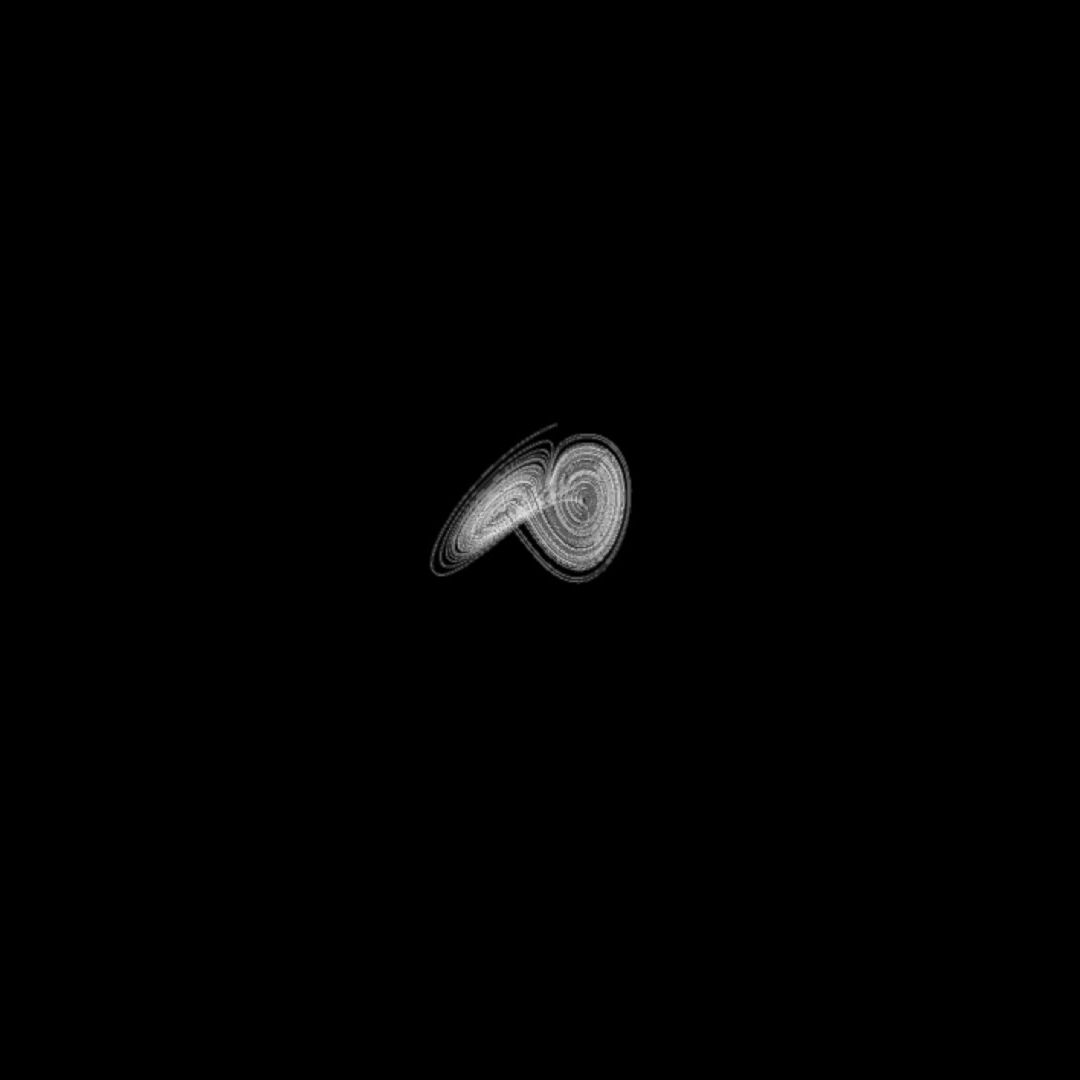
import peasy.*;
import ddf.minim.analysis.*;
import ddf.minim.*;
// Declare and construct four objects
Minim minim;
AudioPlayer test;
FFT fft;
PeasyCam cam;
// Declare three global variables as initial location
float x = 0.01;
float y = 0;
float z = 0;
// Declare three global variables according to the equation
float a = 10;
float b = 28;
float c = 8.0 / 3.0;
float xoff = 0.0;
// An array of points
ArrayList<PVector> points = new ArrayList<PVector>();
void setup() {
size(1280, 720, P3D);
// Draws all geometry with smooth (anti-aliased) edges at level two
smooth(2);
// Initialize the object
minim = new Minim(this);
// Specify that we want the audio buffers of the AudioPlayer
// to be 1024 samples long because our FFT needs to have
// a power-of-two buffer size and this is a good size.
test = minim.loadFile("final.mp3", 1024);
// Create an FFT object that has a time-domain buffer
// the same size as test's sample buffer
// Note that this needs to be a power of two
// and that it means the size of the spectrum will be half as large.
fft = new FFT( test.bufferSize(), test.sampleRate() );
// Initialize the object
cam = new PeasyCam(this, 500);
// Loop the song
test.loop();
}
void draw() {
// Clean the background in order to present new patterns
background(0);
// Perform a forward FFT on the samples in test's mix buffer,
// which contains the mix of both the left and right channels of the file
fft.forward( test.mix );
// Re-maps the variables according to the amplitude of the requested frequency band 7
float w = map(fft.getBand(7), 0, 200, 2, 10);
// Initialize the variables according to the equation
float dt = 0.01;
float dx = (a * (y - x)) * dt;
float dy = (x * (b - z) - y) * dt;
float dz = (x * y - c * z) * dt;
// The location of the points
x = x + dx;
y = y + dy;
z = z + dz;
// Add points from the array
points.add(new PVector(x, y, z));
// Reset the initial point
translate(0, 0, -80);
// Scale its size according to the amlitude of the song
scale(w);
// Set the transparency of stroke according to the amplitude of the requested frequency band i in spectrum size
float alpha = map(fft.getBand(7), 0, 100, 100, 255);
stroke(255, alpha);
strokeWeight(0.5);
noFill();
// Draw the points and connect them together
beginShape();
for (PVector v : points) {
vertex(v.x, v.y, v.z);
}
endShape();
}