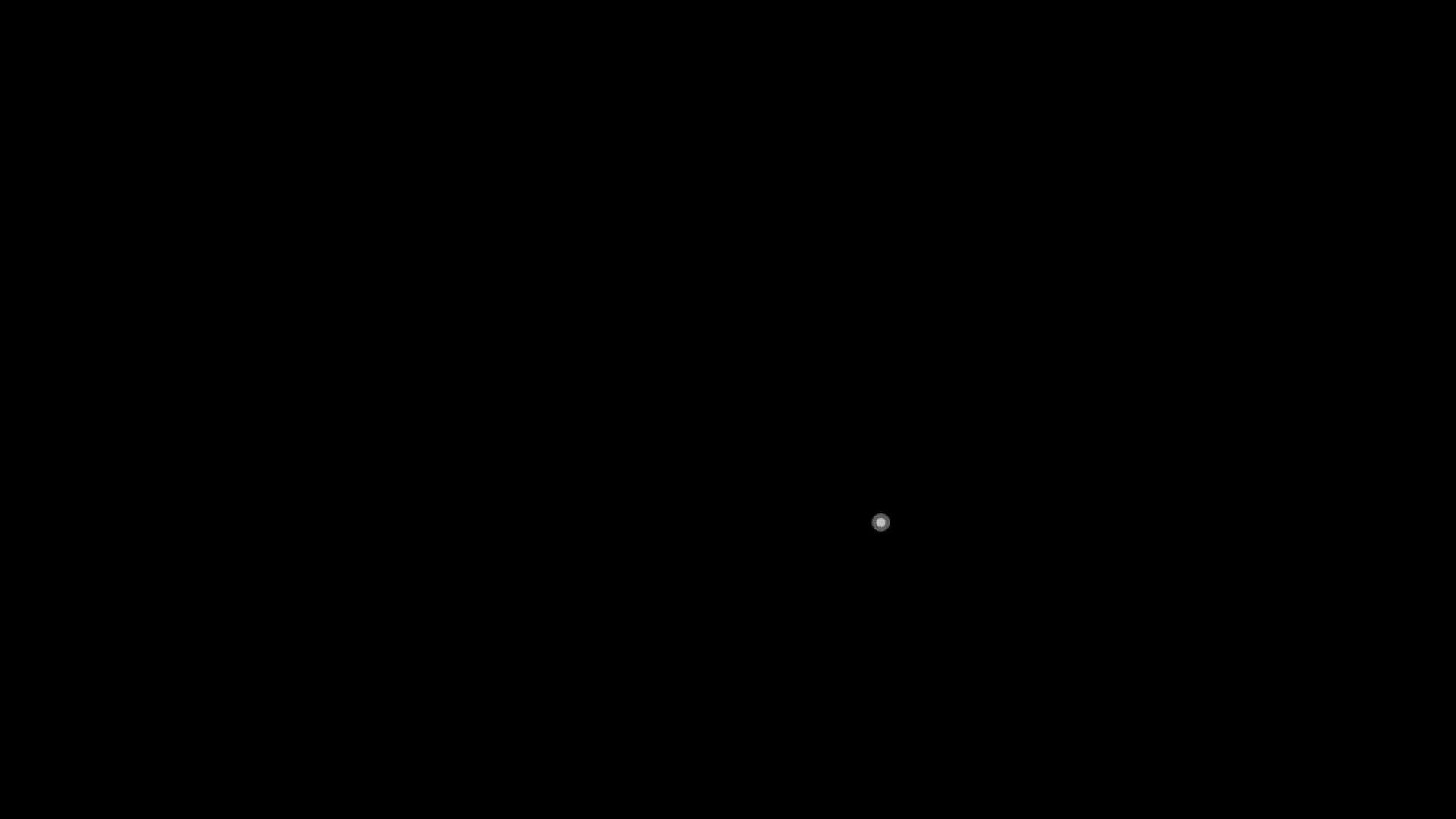
import ddf.minim.analysis.*;
import ddf.minim.*;
// Declare and construct three objects
Minim minim;
AudioPlayer test;
FFT fft;
// Declare two global variables
int n = 0;
int c = 20;
void setup() {
size(1280, 720);
// Draws all geometry with smooth (anti-aliased) edges at level two
smooth(2);
// Initialize the objects
minim = new Minim(this);
// Specify that we want the audio buffers of the AudioPlayer
// to be 1024 samples long because our FFT needs to have
// a power-of-two buffer size and this is a good size.
test = minim.loadFile("final.mp3", 1024);
// Create an FFT object that has a time-domain buffer
// the same size as test's sample buffer
// Note that this needs to be a power of two
// and that it means the size of the spectrum will be half as large.
fft = new FFT(test.bufferSize(), test.sampleRate());
// Play the song
test.play();
}
void draw() {
// Clean the background in order to present new patterns
background(0);
// Perform a forward FFT on the samples in test's mix buffer,
// which contains the mix of both the left and right channels of the file
fft.forward(test.mix);
// Set the variables according to the equation
float a = n * 137.5;
float r = c * sqrt(n);
// Draw ellipses in a polar coordinate system and set them in the center
float x = r * cos(a) + width / 2;
float y = r * sin(a) + height / 2;
// Re-maps the diameter from 0 to 200 according to the amplitude of the requested frequency band 7
float w = map(fft.getBand(7), 0, 100, 0, 200);
// Re-maps the alpha from 100 to 255 according to the amplitude of the requested frequency band 7
float alpha = map(fft.getBand(7), 0, 100, 100, 255);
// Set ellipseMode to RADIUS
ellipseMode(RADIUS);
noStroke();
// Draw ellipses in larger size with higher alpha
fill(255, alpha);
ellipse(x, y, w, w);
// Draw ellipses in smaller size with lower alpha
fill(255, alpha * 3/2);
ellipse(x, y, w / 2, w / 2);
// When the width of ellipses is greater than 10, increase the variable n by 1
if (w > 10) {
n++;
}
// When the value of n is greater than 100, set it back to 0
if (n > 100) {
n=0;
}
}