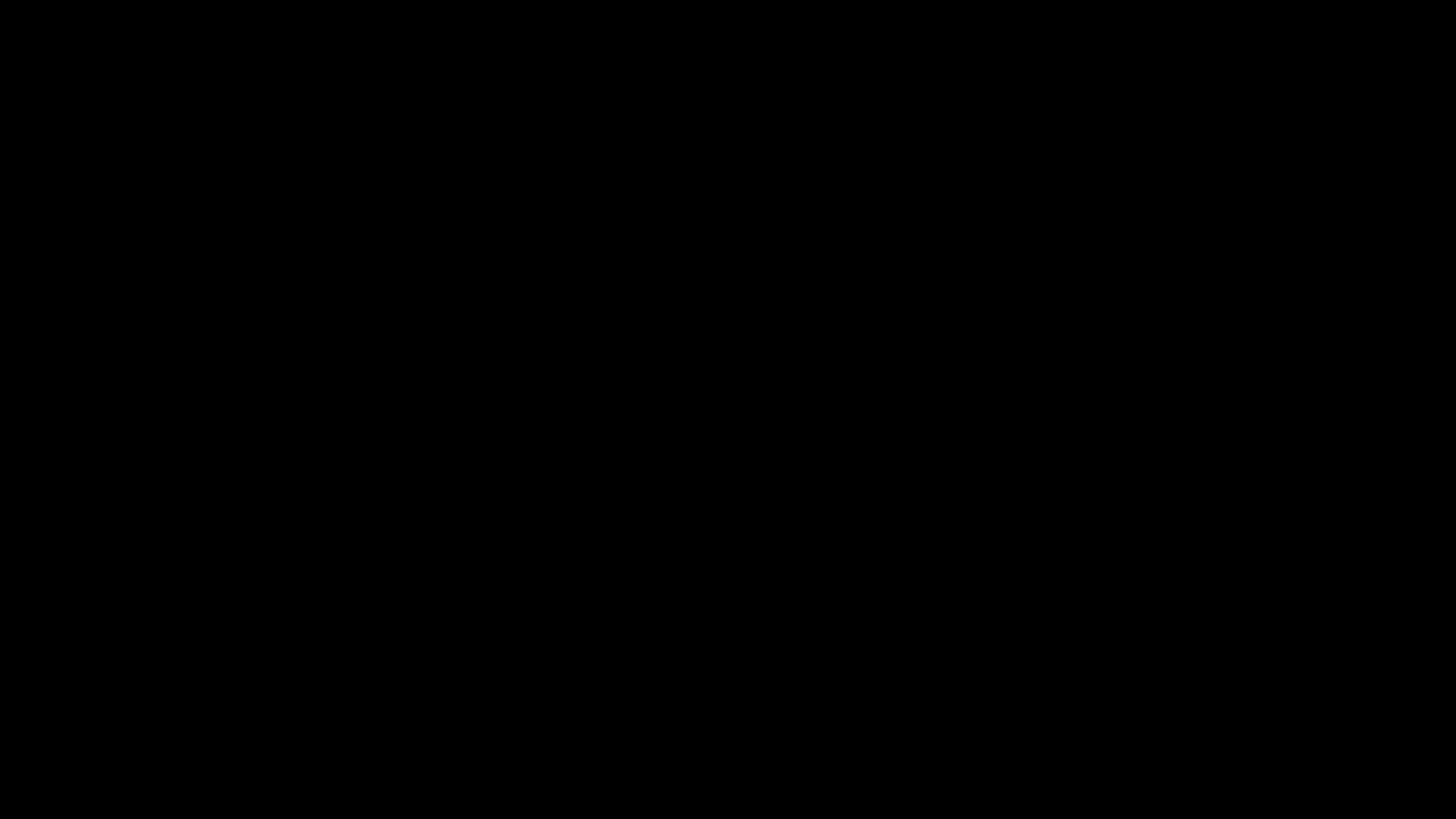
import ddf.minim.*;
import ddf.minim.analysis.*;
// Declare and construct three objects
Minim minim;
AudioPlayer test;
BeatDetect beat;
// Declare a global variable that is the diameter of a ellipse
float eRadius;
void setup() {
size(1280, 720);
// Draws all geometry with smooth (anti-aliased) edges at level two
smooth(2);
// Initialize the objects
minim = new Minim(this);
// Load the song
test = minim.loadFile("final.mp3", 1024);
// A beat detection object song SOUND_ENERGY mode with a sensitivity of 10 milliseconds
beat = new BeatDetect();
// Play the song
test.play();
// Set ellipseMode to RADIUS and initialize the variable into zero
ellipseMode(RADIUS);
eRadius = 0;
}
void draw() {
// Clean the background in order to present new patterns
background(0);
// Analyze the samples in buffer
beat.detect(test.mix);
// Re-maps the diameter from 0 to 255
float w = map(eRadius, 0, 80, 0, 255);
noFill();
stroke(255);
// When a beat has been detected, it returns true and draw a ellipse.
if ( beat.isOnset() ) eRadius = 80;
ellipse(width / 2, height / 2, w, w);
// A ellipse decreases its diameter each time
eRadius *= 0.7;
}